Bryan Mayland
TVWBB Hall of Fame
A long time ago someone had an idea to make a giant 7-segment LED display panel that showed the temperatures from their HeaterMeter in a standalone unit. They never did, and after I priced checked bigger LEDs ($3-5 per digit) I cheaped out and didn't want to make one either. I still had it in the back of my mind and though and I thought it would be fun to put one together.
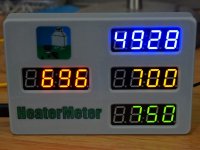
This is a wifi-connected display that updates in real-time just like the web page does. Of course, the LED displays I bought were the "clock display" variety and not the "decimal display" variety so only the smartest geniuses in the world can decipher what temperatures it is showing. Can you?
I'm publishing the source code for this along with the Arduino library that makes integrating whatever you want easy as can be
I've also got a video of it doing what it does
What sets the library apart from most "Display a website's information" programs is that this doesn't poll the HeaterMeter to get the data, it uses the same mechanism that the website does to stream the data in real-time. The updates come as fast as the HeaterMeter puts them out. I'm just displaying the temperatures, but the library also has the PID/fan/servo output, alarm information, and probe names.
I haven't uploaded it to GitHub yet but it will be there in the next day or two. This project took a day start to finish and cost about $11 in parts from China. If you've got an idea for how you'd like to display the live HeaterMeter data in your own special way, it will take you almost no time to get started by just using the library. It doesn't support mDNS discovery because I've found that the mDNS implementation we use is horribly flawed and I need to get it fixed upstream, but maybe we don't even need that.
What can you do with this?!
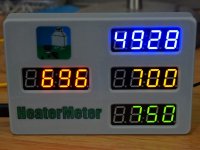
This is a wifi-connected display that updates in real-time just like the web page does. Of course, the LED displays I bought were the "clock display" variety and not the "decimal display" variety so only the smartest geniuses in the world can decipher what temperatures it is showing. Can you?
I'm publishing the source code for this along with the Arduino library that makes integrating whatever you want easy as can be
Code:
#include <HeaterMeterClient.h>
#define WIFI_SSID "network"
#define WIFI_PASSWORD "password"
#define HEATERMEATER_IP "192.168.2.54"
static HeaterMeterClient hm(HEATERMEATER_IP);
static void onHmStatus()
{
// Called every 1-5s as new data comes pushed
Serial.print(hm.state.Probes[0].Name);
Serial.print('=');
Serial.println(hm.state.Probes[0].Temperature);
}
void setup()
{
WiFi.mode(WIFI_STA);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.begin(115200);
hm.onHmStatus = &onHmStatus;
}
void loop()
{
hm.update();
}
I've also got a video of it doing what it does
What sets the library apart from most "Display a website's information" programs is that this doesn't poll the HeaterMeter to get the data, it uses the same mechanism that the website does to stream the data in real-time. The updates come as fast as the HeaterMeter puts them out. I'm just displaying the temperatures, but the library also has the PID/fan/servo output, alarm information, and probe names.
I haven't uploaded it to GitHub yet but it will be there in the next day or two. This project took a day start to finish and cost about $11 in parts from China. If you've got an idea for how you'd like to display the live HeaterMeter data in your own special way, it will take you almost no time to get started by just using the library. It doesn't support mDNS discovery because I've found that the mDNS implementation we use is horribly flawed and I need to get it fixed upstream, but maybe we don't even need that.
What can you do with this?!
Last edited: